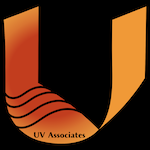
iOS Scholar's Blog
iOS Development Tutorials, Tips & Tricks and more
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
for..< in Loop in Swift Programming Language
for loop in Swift Programming Language
Iterating over Ranges, Collections and more
Note: Updated for Swift 3
Apple's new programming language Swift, has a for-in loop.
(There was a classic for loop statement available earlier, but has been removed in Swift 3.0)
for-in Loop for Iterating over Ranges, Collections etc.
This construct is not new, albeit interesting - many other languages have a similar construct to iterate over a list of values, or a range or a collection.
Iterating over Open and Closed Ranges
Here is a simple form of the for-in loop which iterates over a range:
for i in 1...5
{
print ("Four multiplied by \(i) results in \(i*4)" )
}
The above loop prints 5 multiples of 4 by iterating over the “open range” 1 to 5. An open range will include both the upper and lower values. In contrast, the next example uses the "closed range" operator (..<) which includes the lower, but not the upper value.
Important: The iterator used in for-in loop is not accessible outside the body of the loop. Even if you declare a variable before the for-in loop, and use an iterator with same name, iterator would still be a different variable, with a temporary scope - local to the body of the loop.
var i=0
for i in 1..<10
{
print("Number \(i)")
}
print(i)
// will print 0
The i which gets printed is the global i declared before the body of the loop. It’s not the iterator - that’s never accessible in case of for-in loop. This isn’t the case with the traditional for loop. If you have declared a variable outside the body of the loop, and use that variable in the “initialization” and the “increment” statements, its value is retained, and is usable after the loop completes execution.
Note that in the above examples, we’re using the iterator i inside the loop. If the iterator is of no use to us inside the body of the loop, we can simply use an underscore:
var twoToThePowerN = 2
var n = 5
for _ in 1..<n {
twoToThePowerN *= 2
}
print(twoToThePowerN)
//prints 32
In the above example, we don’t need access to the current value of the iterator, all we want is the loop should be executed n-1 times, thus we use the close range 1..
Iterating over an Array’s Elements
Here is another example, this one iterates over elements of an array literal:
for element in ["Ray", 4, 3, 1.3]
{
print (i)
}
The array literal above is of type AnyObject. Thus, very few operations common to all objects like print would be possible for the elements of this array. (However, we may check the type and then downcast to perform more operations specific to a particular type.)
Iterating over Keys or Values or both of a Dictionary
var releasedSequels = ["Xmen":7, "LOTR":4, "Matrix":3, "Spider-Man":4]
var names = ""
for (key, _) in releasedSequels {
names = names + key + " "
}
print(names)
// Prints: Matrix Xmen LOTR Spider-Man
Since we don’t need the number of sequels, we use an underscore to express our only interest which is printing names of the great movies.
Conclusion
So this was the for loop construct of the Swift programming Language. A little familiar, and refreshing too. Get hang of it, wish you merry looping around in the Playground.
----
*Seriously, not requiring semicolons after each statement is spoiling me. I execute programs in C/C++ after having fun in Swift, only to spend a lot of time inserting the missing semicolons :D
** The following image uses println, which doesn't work in the new version, use print instead.